An automated new pending custom post type from user filled form
This project was done for EnergyComIL, to build a companies database.
When a user fills the form to join the database – a new post is created automatically with the details entered in via the joining form. The post is created with a “pending approval” status. The details includes custom fields, the post thumbnail image and the post category.
After manual approval (as asked for by the client), the company is added to the database.
The database is searchable and filtered with Ajax.
This post is a custom post type, with custom taxonomies (non-hierarchal).
Here is some of the code i’ve written to create this:
//Handle the form after it is sent with Elementor API webhook
function send_custom_webhook( $record, $handler ) {
// Check that this is the right form on the site
if ( 'b2bform' !== $form_name ) {
return;
}
$raw_fields = $record->get( 'fields' );
$fields = [];
foreach ( $raw_fields as $id => $field ) {
$fields[ $id ] = $field['value'];
}
// Create post object
$my_post = array(
'post_author' => 1,
'post_content' => $fields['tech_description'],
'post_type' => 'company',
'post_title' => $fields['company_name'],
'tax_input' => array('company_category' => $fields['company_category']),
'post_status' => 'pending',
);
//create the post
$postID = wp_insert_post( $my_post );
//create post thumbnail
$image_url = $fields['logo']; // Define the image URL here
$image_name = str_replace('https://energycom.org.il/wp-content/uploads/elementor/forms/', '', $fields['logo']);
$upload_dir = wp_upload_dir(); // Set upload folder
$image_data = file_get_contents($image_url); // Get image data
$unique_file_name = wp_unique_filename( $upload_dir['path'], $image_name );
// Generate unique name
$filename = basename( $unique_file_name ); // Create image file name
// Check folder permission and define file location
if( wp_mkdir_p( $upload_dir['path'] ) ) {
$file = $upload_dir['path'] . '/' . $filename;
} else {
$file = $upload_dir['basedir'] . '/' . $filename;
}
// Create the image file on the server
file_put_contents( $file, $image_data );
// Check image file type
$wp_filetype = wp_check_filetype( $filename, null );
// Set attachment data
$attachment = array(
'post_mime_type' => $wp_filetype['type'],
'post_title' => sanitize_file_name( $filename ),
'post_content' => '',
'post_status' => 'inherit'
);
// Create the attachment
$attach_id = wp_insert_attachment( $attachment, $file, $postID );
// Include image.php
require_once(ABSPATH . 'wp-admin/includes/image.php');
// Define attachment metadata
$attach_data = wp_generate_attachment_metadata( $attach_id, $file );
// Assign metadata to attachment
wp_update_attachment_metadata( $attach_id, $attach_data );
// And finally assign featured image to post
set_post_thumbnail( $postID, $attach_id );
}
add_action( 'elementor_pro/forms/new_record', 'send_custom_webhook' , 10, 2);
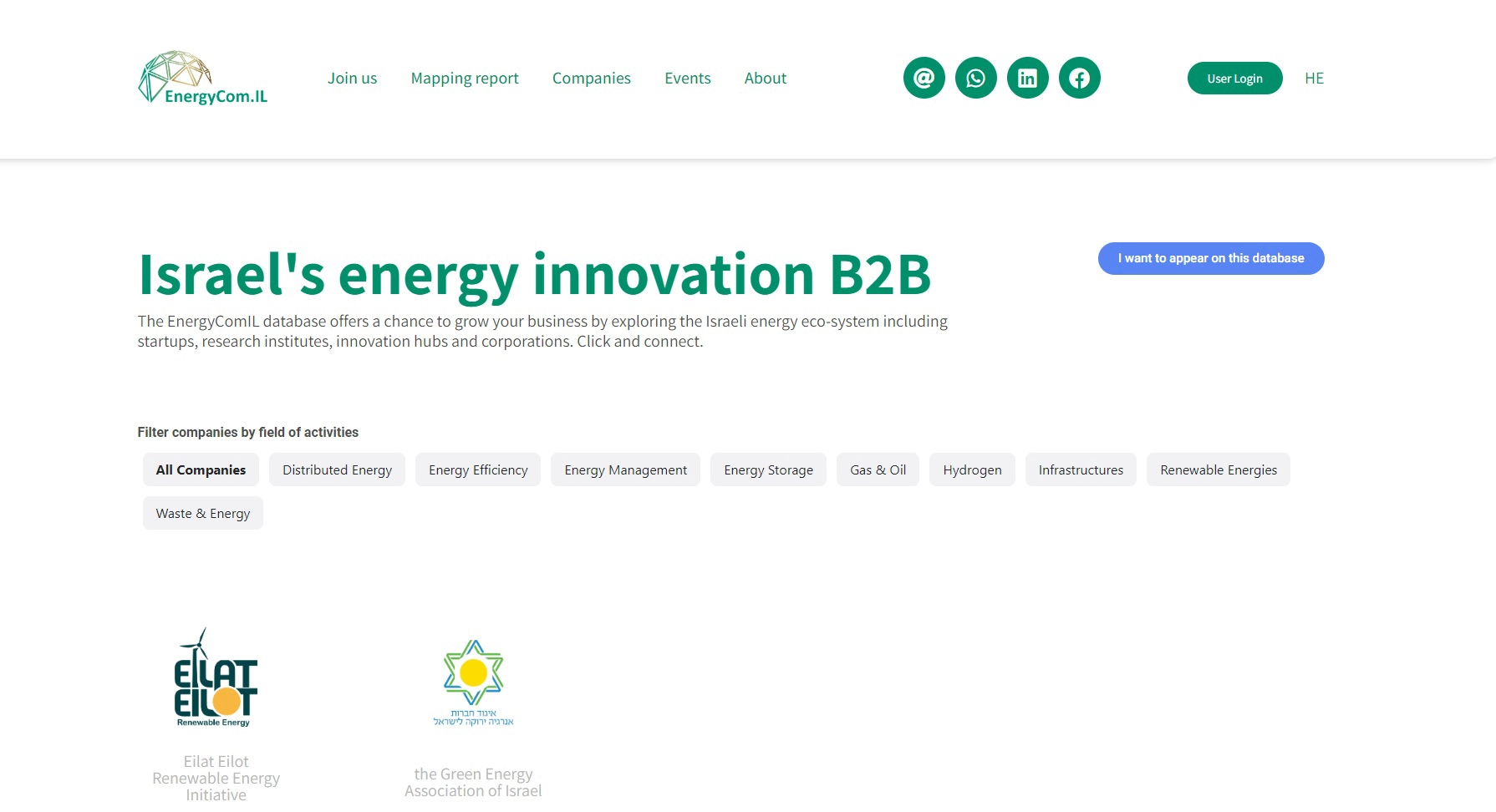